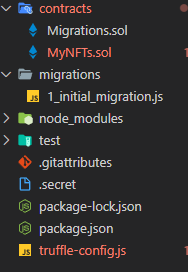
ERC-721 배포하기
세팅
mkdir 폴더이름
truffle init
npm init
npm i truffle
npm install @openzeppelin/contracts
미그레이션에 코드추가하기
const Migrations = artifacts.require('Migrations');
const MyNFTs = artifacts.require('MyNFTs.sol'); // MyNFTs.sol 파일 추가
module.exports = function (deployer) {
deployer.deploy(Migrations);
deployer.deploy(MyNFTs); // MyNFTs를 배포에 추가
};
nft 컨트랙트파일생성
//Contract based on [https://docs.openzeppelin.com/contracts/3.x/erc721](https://docs.openzeppelin.com/contracts/3.x/erc721)
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.7;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/utils/Counters.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol";
contract MyNFTs is ERC721URIStorage, Ownable {
using Counters for Counters.Counter;
Counters.Counter private _tokenIds;
constructor() public ERC721("MyNFTs", "MNFT") {}
function mintNFT(string memory tokenURI)
public
onlyOwner
returns (uint256)
{
_tokenIds.increment();
uint256 newItemId = _tokenIds.current();
_mint(msg.sender, newItemId);
_setTokenURI(newItemId, tokenURI);
return newItemId;
}
}
truffle.config.js 파일설정
networks: {
// Useful for testing. The `development` name is special - truffle uses it by default
// if it's defined here and no other network is specified at the command line.
// You should run a client (like ganache, geth, or parity) in a separate terminal
// tab if you use this network and you must also set the `host`, `port` and `network_id`
// options below to some value.
//
ganache: {
host: "127.0.0.1", // Localhost (default: none)
port: 7545, // Standard Ethereum port (default: none)
network_id: "*", // Any network (default: none)
},
//
// An additional network, but with some advanced options…
// advanced: {
// port: 8777, // Custom port
// network_id: 1342, // Custom network
// gas: 8500000, // Gas sent with each transaction (default: ~6700000)
// gasPrice: 20000000000, // 20 gwei (in wei) (default: 100 gwei)
// from: <address>, // Account to send transactions from (default: accounts[0])
// websocket: true // Enable EventEmitter interface for web3 (default: false)
// },
//
// Useful for deploying to a public network.
// Note: It's important to wrap the provider as a function to ensure truffle uses a new provider every time.
// ropsten: {
// provider: () => new HDWalletProvider(mnemonic, `https://ropsten.infura.io/v3/YOUR-PROJECT-ID`),
// network_id: 3, // Ropsten's id
// gas: 5500000, // Ropsten has a lower block limit than mainnet
// confirmations: 2, // # of confirmations to wait between deployments. (default: 0)
// timeoutBlocks: 200, // # of blocks before a deployment times out (minimum/default: 50)
// skipDryRun: true // Skip dry run before migrations? (default: false for public nets )
// },
//
// Useful for private networks
// private: {
// provider: () => new HDWalletProvider(mnemonic, `https://network.io`),
// network_id: 2111, // This network is yours, in the cloud.
// production: true // Treats this network as if it was a public net. (default: false)
// }
},
// Set default mocha options here, use special reporters, etc.
mocha: {
// timeout: 100000
},
// Configure your compilers
compilers: {
solc: {
version: "0.8.7", // Fetch exact version from solc-bin (default: truffle's version)
// docker: true, // Use "0.5.1" you've installed locally with docker (default: false)
// settings: { // See the solidity docs for advice about optimization and evmVersion
// optimizer: {
// enabled: false,
// runs: 200
// },
evmVersion: "byzantium"
// }
}
},
오픈제플린으로 컴파일 및 가나슈네트워크에 연결하기
truffle migrate --compile-all --network ganache
미그레이션진행
가나슈 네트워크에 연결된모습
배포가 잘됐는지 instance 명령어로 확인
토큰url도 잘받아왔는지 확인
내가 만든 nft를 배포하고싶다면?
const HDWalletProvider = require('@truffle/hdwallet-provider');
//
const fs = require('fs');
const mnemonic = fs.readFileSync(".secret").toString().trim();
config파일 고쳐주고 .secret 파일에 지갑개인키 넣어서 진행해주자
Keep (장점, 유지할 점)
- 트러플과 오픈제플린 환경을 통해 nft를 손쉽게배포할수있었다.
- 파일관련 문제들을 빠르게 구글링해서 문제점을 찾아내서해결했다
Problem (단점, 변경 또는 버릴 점)
- solidiy 버젼이 충돌하게되면 컴파일에러가 자주난다 잘확인해줘야한다.
Try (시도할 점, 앞으로의 행동)
nft 배포하는 사이트를 만들어보면 좀더 친숙하게 다가올것같다 만들것